11. Product List - Pagination
Contents
Through paging techniques, we can only show some of the products.
Add More Products into Database
Run the following SQL inside the database:
|
|
Create Pagination Utilities
Create PageHelper
- Right click common/src/main/java/co.dongchen.shop.common/util directory: New > Java Class
- Fill in “PageHelper”
- Click “OK” button
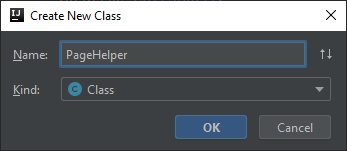
Copy and paste the following content into PageHelper.java:
|
|
Create Pagination
- Right click common/src/main/java directory: New > Package
- Fill in “co.dongchen.shop.common.util.pager”
- Click “OK” button
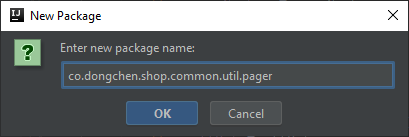
- Right click common/src/main/java/co.dongchen.shop.common/util/pager directory: New > Java Class
- Fill in “Pagination”
- Click “OK” button
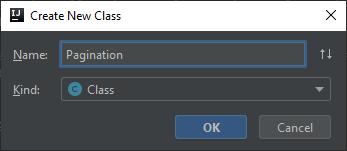
Copy and paste the following content into Pagination.java:
|
|
Create PagingData
- Right click common/src/main/java/co.dongchen.shop.common/util/pager directory: New > Java Class
- Fill in “PagingData”
- Click “OK” button
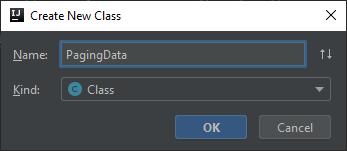
Copy and paste the following content into PagingData.java:
|
|
Update Product Mapper
Update ProductMapper interface
Copy and paste the following content into ProductMapper.java under the service/src/main/java/co.dongchen.shop/mapper directory:
|
|
Update product.xml
Copy and paste the following content into product.xml under the service/src/resources/mapper directory:
|
|
Update ProductService class
Copy and paste the following content into ProductService.java under the service/src/java/co.dongchen.shop/service directory:
|
|
Update ProductController class
Copy and paste the following content into ProductController.java under the controller/src/java/co.dongchen.shop/controller directory:
|
|
Update common.ftl in Controller Module
Append the following content into common.ftl template:
|
|
Create common.js in Controller Module
- Right click controller/src/main/resources directory: New > Directory
- Fill in “static/static/common/js”
- Click “OK” button
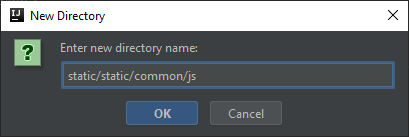
- Right click static.static.common.js directory: New > File
- Fill in “common.js”
- Click “OK” button
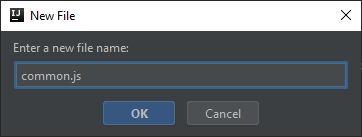
Copy and paste the following content into common.js:
|
|
Create pagination.ftl in Controller Module
- Right click controller/src/main/resources/templates directory: New > Directory
- Fill in “product”
- Click “OK” button
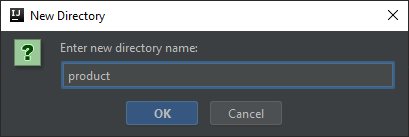
- Right click product directory: New > File
- Fill in “pagination.ftl”
- Click “OK” button
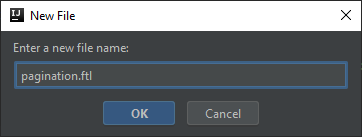
Copy and paste the following content into pagination.ftl:
|
|
Remove a Method from FreeMarkerController
Remove the following method from FreeMarkerController:
|
|
See here for more information about how to insert data into database.
Verify
Run the App
|
|
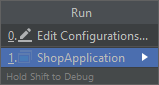
Choose the correspondent option and press enter.
View Product List Page in the Browser
|
|
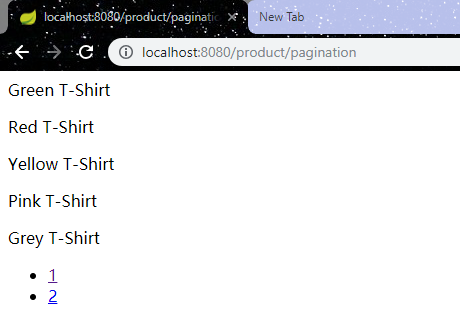
Click linkage number 2 to the second page:
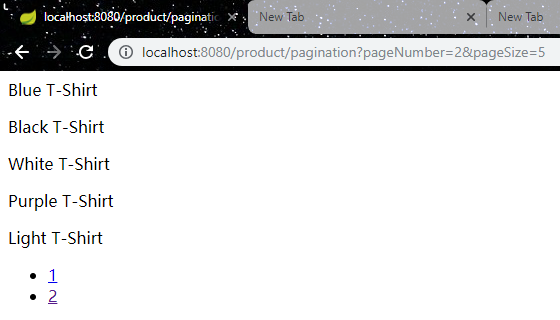
Author Dong Chen
LastMod Sat May 4 2019