2. Database Access - MyBatis and MariaDB 10.x
Contents
We first need to install a MariaDB 10.x database locally, and then access the database through MyBatis configuration.
Data Preparation
Install Database Locally
Since I also develop PHP projects on my Windows 10 desktop PC, I’ll use the MariaDB 10.x that comes with the WAMPSERVER for convenience:
Create Database and Table
Run following SQL script in MySQL or MariaDB database console:
|
|
After executing the above SQL scripts, you may see the following results:
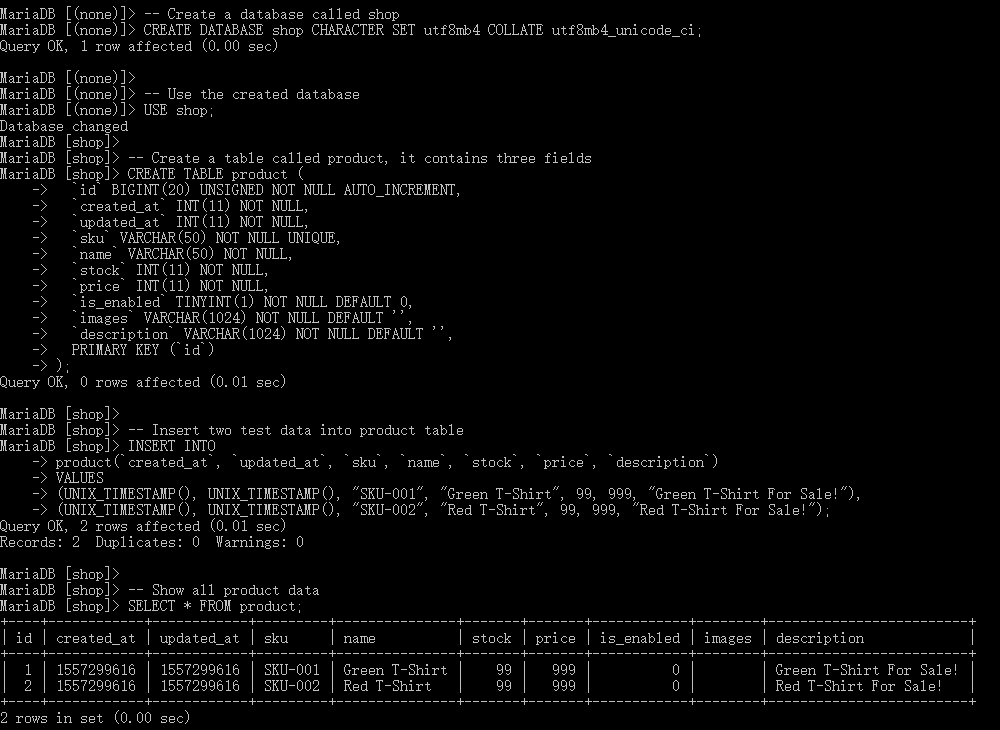
Dependencies and Configurations
Add MyBatis and MySQL Dependencies
Add MyBatis dependency into pom.xml:
|
|
Add MySQL dependency into pom.xml:
|
|
Add JDBC Data Source
Add JDBC Data Source into application.properties
|
|
Add MyBatis Configurations
Add MyBatis configuration path into application.properties:
|
|
- Right click resources directory: New > Directory
- Fill in: mybatis
- Click “OK” button
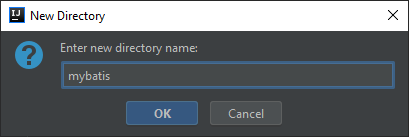
- Right click mybatis directory: New > File
- Fill in: mybatis-config.xml
- Click “OK” button
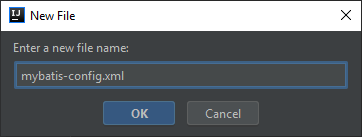
Copy and paste the following content into mybatis-config.xml:
|
|
Model View Controller (MVC)
Create Model
- Right click java directory: New > Package
- Fill in: co.dongchen.shop.common.model
- Click “OK” button
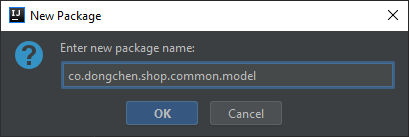
- Right click co.dongchen.shop.common.model directory: New > Java Class
- Fill in: Product
- Click “OK” button
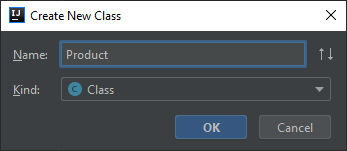
Copy and paste the following content into Product.java:
|
|
Create Mapper Interface
- Right click java directory: New > Package
- Fill in: co.dongchen.shop.mapper
- Click “OK” button
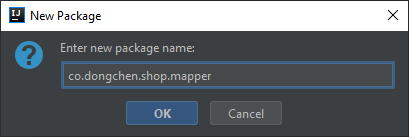
- Right click co.dongchen.shop.mapper directory: New > Java Class
- Fill in: ProductMapper
- Change Kind to: Interface
- Click “OK” button
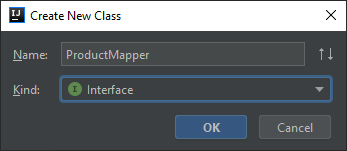
Copy and paste the following content into ProductMapper.java:
|
|
Create Mapper XML
- Right click resources directory: New > Directory
- Fill in: mapper
- Click “OK” button
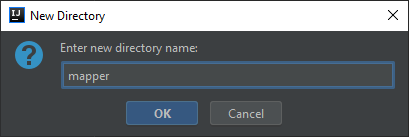
- Right click mapper directory: New > File
- Fill in: product.xml
- Click “OK” button
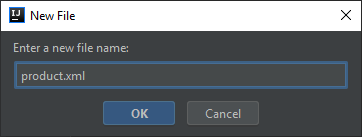
Copy and paste the following content into product.xml:
|
|
Create Service
- Right click java directory: New > Package
- Fill in: co.dongchen.shop.service
- Click “OK” button
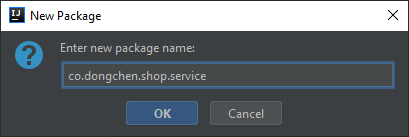
- Right click co.dongchen.shop.service directory: New > Java Class
- Fill in: ProductService
- Click “OK” button
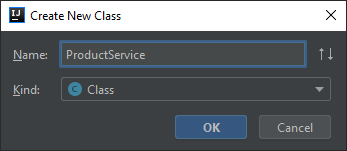
Copy and paste the following content into ProductService.java:
|
|
Create Controller
- Right click java directory: New > Package
- Fill in: co.dongchen.shop.controller
- Click “OK” button
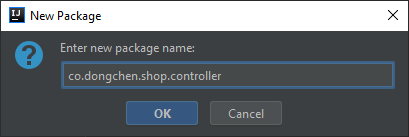
- Right click co.dongchen.shop.controller directory: New > Java Class
- Fill in: ProductController
- Click “OK” button
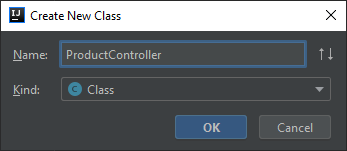
Copy and paste the following content into ProductController.java:
|
|
Verify
Run the App
|
|
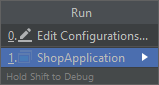
Choose the correspondent option and press enter.
View Products in the Browser
|
|
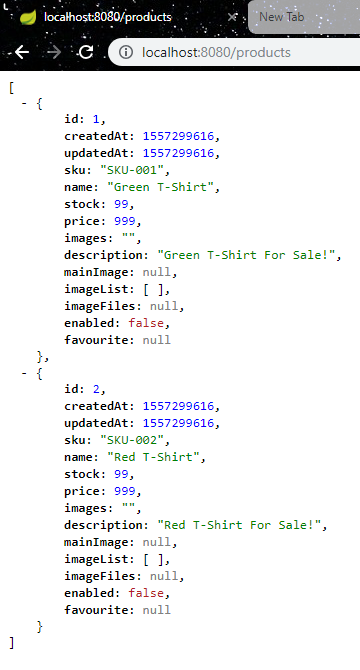
References Wampserver, mybatis-spring-boot-autoconfigure, Maven Repository: MySQL Connector/J » 8.0.15
Author Dong Chen
LastMod Thu Apr 25 2019