8. User Login - Spring Security
Contents
Registered users can log into the system through a login interface.
Add Attribute into application.properties file
Add the image path on the file server into application.properties file:
|
|
Add Property into User Service
Add following property into the UserService.java file under the service/src/main/java/co.dongchen.shop/service directory:
|
|
Add Methods into the User Service
Add following methods into the UserService.java file under the service/src/main/java/co.dongchen.shop/service directory:
|
|
Create Constants Class
- Right click common/src/main/java directory: New > Package
- Fill in “co.dongchen.shop.common.constant”
- Click “OK” button
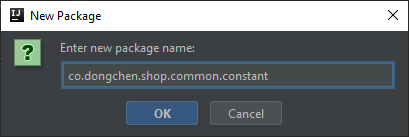
- Right click common/src/main/java/co.dongchen.shop.common/constant directory: New > Java Class
- Fill in “Constants”
- Click “OK” button
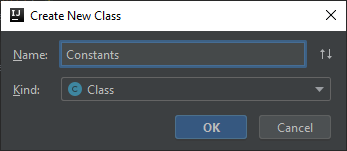
Copy and paste the following content into Constants.java:
|
|
Add Method into the User Controller
Add following methods into the UserController.java file under the controller/src/main/java/co.dongchen.shop/controller directory:
|
|
Create Templates
Create Login Template
- Right click controller/src/main/resources/templates/user directory: New > File
- Fill in “login.ftl”
- Click “OK” button
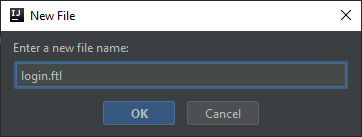
Copy and paste the following content into login.ftl:
|
|
Create Login Succeeded Template
- Right click controller/src/main/resources/templates/user directory: New > File
- Fill in “login_succeeded.ftl”
- Click “OK” button
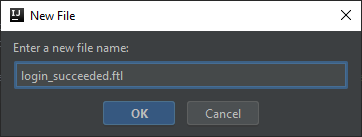
Copy and paste the following content into login_succeeded.ftl:
|
|
Verify
Run the App
|
|
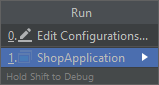
Choose the correspondent option and press enter.
View Login Page in the Browser
|
|
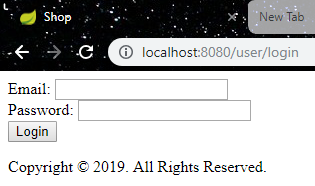
- Fill in the Information and click login:
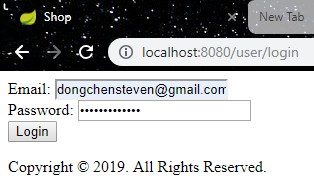
- We will see a login succeeded page:
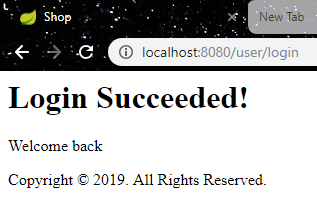
Author Dong Chen
LastMod Wed May 1 2019