31. Bash Shell - Expansions: Command, Arithmetic, Process Substitution
Contents
Linux provides us with some expansions for command, arithmetic, addition and subtraction, comparison, ternary, assignment, and process substitution operations.
Command
We can execute the output as a command by using the $() or `` symbols.
Let’s use the $() symbols to do this first:
|
|
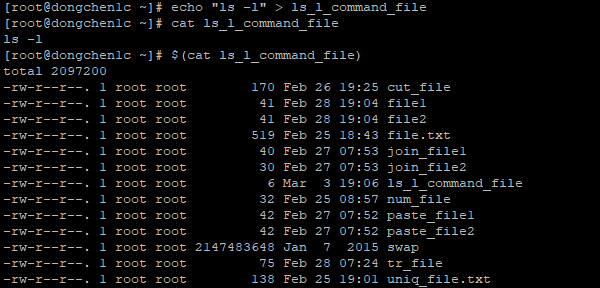
Alternatively, we can use backtick symbols to achieve the same effect:
|
|
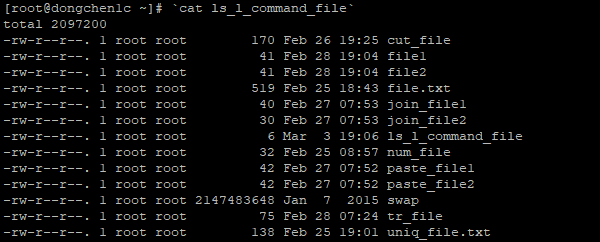
Arithmetic
We can use arithmetic expansion to quickly calculate numerical values.
Some of the operators we can use are:
Operator | Meaning |
---|---|
VARIABLE++ VARIABLE‑‑ | self increasing or deceasing after the variable is obtained |
++VARIABLE ‑‑VARIABLE | self increasing or deceasing before the variable is obtained |
- + | negative or positive number |
! ~ | logical or bitwise negation |
** | exponentiation |
* / % | multiplication, division, remainder |
+ - | addition, subtraction |
<= >= < > | less than equals, greater than equals, less than, greater than |
== != | equal or not equal |
expr ? expr : expr | ternary operator |
= *= /= %= += -= <<= >>= &= ^= | assign the operation result to a variable |
Addition and Subtraction
|
|

Prints addition and subtraction results
Comparison
|
|
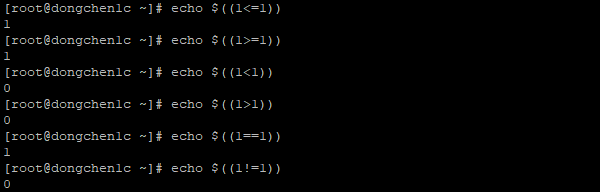
Prints comparison results
Ternary Operator
Positive and negative numbers are true, only zero is false
|
|

Prints true or false of the ternary operation result
Assignment Operator
|
|

Prints assignment operation results
Process Substitution
We can use the <() symbols to use the result of the command output as a file:
|
|
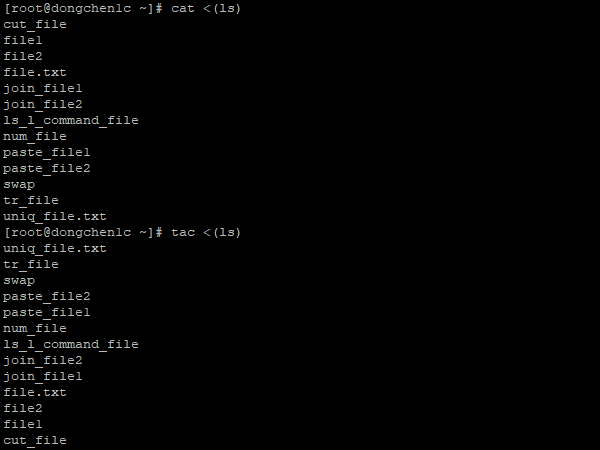
Treat ls results as a file and print it with cat and tac commands
References 3.5.4 Command Substitution, 3.5.5 Arithmetic Expansion, 6.5 Shell Arithmetic, 3.5.6 Process Substitution
Author Dong Chen
LastMod Mon Mar 4 2019