38. Processes - Running Jobs Management
Contents
In linux, each running program will create at least one process in memory, and each process will be assigned a process ID, we can use the ID of the process to do the corresponding operation of a running program.
Prepare the Necessary Shell Script
Let’s create a shell script program that doesn’t exit itself to do the demonstration:
|
|

We have a shell script ready for infinite loop operations
We’re not going to talk too much about the shell script right now. The only thing we need to know is that this script doesn’t exit itself after it runs.
Execute Scripts in the Background
Let’s execute two scripts one at a time and let them run constantly in the background:
|
|

Execute two scripts with the bash command and keep them running in the background
List All Programs Running in the Background
We can use the jobs command to see which programs are running in the background.
Some common parameters of the jobs command are:
Option | Meaning |
---|---|
-l | In addition to basic information, list the ID for each process |
-p | List only the ID for each process |
-r | Show only running processes |
-s | Show only processes that stop running |
Let’s take a look at the output of the jobs command without specifying parameters:
|
|

Show all the jobs running in the background
List With Their Process IDs
List the jobs and the process ID for each program with the -l option:
|
|

Show all the jobs and each job's process ID
List the Process IDs Only
List the jobs’ process ID for each program with the -p option:
|
|

Show each job's process ID only
See How the Program is Running in the Background
We can see how the two scripts work through the top command:
|
|
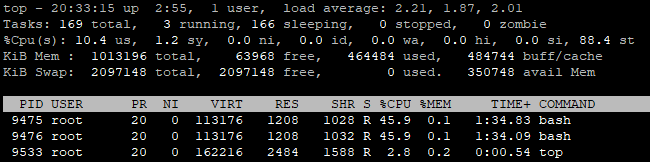
A list of running programs
Through the screenshot, we know that there are three programs currently running, two of which are the two scripts we just ran, and the other is the top command itself.
We also found that both scripts have a common problem, which is that they consume CPU very much during their run-time.
Terminate Running Processes
We can use the kill command to end the life cycle of a running process with some associated signal values.
The kill command sends a TERM(15) signal to a process by default:
|
|
Some common signal options are:
Signal | Meaning |
---|---|
-TERM or -15 | Terminate one or more processes |
-KILL or -9 | Kill one or more processes with results that cannot be captured and ignored |
-HUP or -1 | Hangup one or more processes |
-INT or -2 | Terminal interrupt one or more processes |
-QUIT or -3 | Terminal quit one or more processes |
The kill command has three running results, success, failure, and partial success:
Return Code | Meaning |
---|---|
0 | Termination succeeded |
1 | Termination failed |
64 | Termination partially succeeded (When multiple process IDs are specified) |
Forced Termination of a Running Process
But sometimes situations are special, and some processes cannot be terminated by the default signal.
Then we need to use the KILL(9) signal to terminate the process:
|
|
Unforced Termination of a Running Process
Usually, we would only use the TERM(15) signal to terminate a running process:
|
|
Because the code for this script is relatively simple, so the two processes running based on this script can be terminated using the kill command’s default signal:
|
|

List all jobs after killing the two processes
From the screenshot, we can see that both processes have been killed.
References 7.2 Job Control Builtins, 24 Process control, 24.1 kill: Send a signal to processes, 2.5 Signal specifications, KILL(1)
Author Dong Chen
LastMod Sun Mar 10 2019